What is Encapsulation in Java?
Encapsulation is process of wrapping code and data together into a single unit.
POJO(Plain Old Java Object) class is the best example of fully encapsulated class.
Encapsulation is also known as "Data hiding" because they are protecting data which is prone to change.
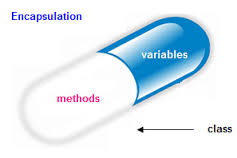
How Is Encapsulation Done in Java?
Encapsulation is implemented in Java using classes, access specifiers, setters and getters.
A class or an interface encapsulates essential features of an object.
Access specifiers (private, public, protected) restrict access to data at different levels.
Setters and getters prevent data from misuse or unwanted changes from other objects.
Example
class Employee{ private String employeeName; public String getEmployeeName() { return employeeName; } public void setEmployeeName(String employeeName) { this.employeeName = employeeName; } public static void main(String[] args){ Employee s=new Employee(); s.setEmployeeName("John"); System.out.println(s.getEmployeeName()); } }Try it Yourself
Why Encapsulation use in Java Code?
Here are some advantages to use encapsulation in Java Code.
- Encapsulated Code is more flexible and easy to change with new requirements.
- By providing only getter and setter method access, you can make the class read only.
- Encapsulation in Java makes unit testing easy.
- A class can have total control over what is stored in its fields. Suppose you want to set the value of marks field i.e. marks should be positive value, than you can write the logic of positive value in setter method.
- Encapsulation also helps to write immutable class in Java which are a good choice in multi-threading environments.
- Encapsulation allows you to change one part of code without affecting other part of code.
Abstraction | Encapsulation |
---|---|
Abstraction solves the problem in the design level. | Encapsulation solves the problem in the implementation level. |
Abstraction is used for hiding the unwanted data and giving relevant data. | Encapsulation means hiding the code and data into a single unit to protect the data from outside world. |
Abstraction lets you focus on what the object does instead of how it does it | Encapsulation means hiding the internal details or mechanics of how an object does something. |
Abstraction- Outer layout, used in terms of design.
For Example:Outer Look of a Mobile Phone, like it has a display screen and keypad buttons to dial a number.
|
Encapsulation- Inner layout, used in terms of implementation.
For Example:- Inner Implementation detail of a Mobile Phone, how keypad button and Display Screen are connect with each other using circuits.
|
Solve this!!
11834.Which of the these is the functionality of ‘Encapsulation’?
Binds together code and data
Using single interface for general class of actions.
Reduce Complexity
All of the mentioned
- Collection
- ArrayList
- LinkedList
- Vector
- HashSet
- Conditional Statements
- Classes and Objects
- Inheritance
- Packages
- Exception Handling
- Threads
- TreeSet
- LinkedHashSet
- HashMap
- TreeMap
- LinkedHashMap
- HashTable
- Iterator and ListIterator
- String
- Scanner
- Array Small Programs
- Introduction
- Variables
- DataTypes
- Operators
- Conditional Statements
- Loop
- Arrays
- Object Oriented Programming
- Classes and Objects
- Access Modifiers
- Methods
- Abstraction
- Constructor
- Packages
- StringBuffer
- Inheritance
- StringBuilder
- Encapsulation
- Java IO
- Polymorphism
- Interface
- Types Of Variables
- Static Keyword
- Final Keyword
- Super Keyword
- This Keyword
- Exception Handling