What is Interface?
An interface in java is a blueprint of a class. It has static constants and abstract methods.
The interface in java is a mechanism to achieve abstraction.
An Java interface cannot contain an implementation of the methods, only the signature (name, parameters) of the method.
It cannot be instantiated just like abstract class.
Why use interface in java?
It is use to achieve fully abstraction.
By using interface we achieve multiple inheritances.
How to create interface in java?
The interface keyword is used to create an interface.
Use implements keyword to implement interface into class.
Syntax
public interface Interface-name{ //Any number of final, static fields //Any number of abstract method declarations }
Example:
interface shape{ public void Draw(); } class circle implements shape{ public void Draw() { System.out.println("Drawing Circle here"); } public static void main(String[] args) { shape c1=new circle(); c1.Draw(); } }Try it Yourself
How to implement multiple inheritance using interface?
We can achieve multiple inheritance using multiple interface by comma separated list after implements keyword.
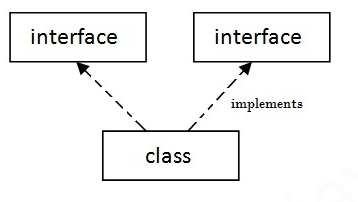
Example:
interface Greeting{ void print(); } interface Welcome{ void show(); } class A1 implements Greeting,Welcome{ public void print(){ System.out.println("This is Greeting"); } public void show(){ System.out.println("This is Welcome"); } public static void main(String[] args){ A1 obj=new A1(); obj.print(); obj.show(); } }Try it Yourself
Example:
interface Greeting{ void print(); } class Welcome{ void show(){ System.out.println("This is Welcome"); } } class A1 extends Welcome implements Greeting{ public void print(){ System.out.println("This is Greeting"); } public static void main(String[] args){ A1 obj=new A1(); obj.print(); obj.show(); } }Try it Yourself
It is Possible to Extending interface?
Yes,An interface can extends another interface like the way to the class extends another class using extends keyword.
The child interface inheritance the method and member variable of parent interface.
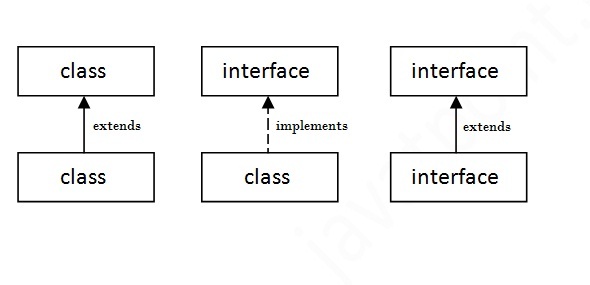
Example:
interface printable{ void print(); } interface showable extends printable{ void show(); } class A2 implements showable{ public void print(){ System.out.println("Hello"); } public void show(){ System.out.println("Welcome"); } public static void main(String[] args){ showable obj=new A2(); obj.print(); obj.show(); } }Try it Yourself
What is marker interface in java and why required?
Marker interface in java is interface with no member variable or methods or in simple word empty interface called marker interface in Java, also known as tagging interface. E.g. Serializable, Clonnable.
Marker interface used to indicate something to compiler or JVM. So if JVM sees a Class is Serializable it done some special operation on it, similar way if JVM sees one Class is implement Clonnable it performs some operation to support cloning.
Example:
Interface MarkerInterface{ }
Solve this!!
interface calculate { void cal(int item); } class display implements calculate { int x; public void cal(int item) { x = item * item; } } class interfaces { public static void main(String args[]) { display arr = new display; arr.x = 0; arr.cal(2); System.out.print(arr.x); } }
- Collection
- ArrayList
- LinkedList
- Vector
- HashSet
- Conditional Statements
- Classes and Objects
- Inheritance
- Packages
- Exception Handling
- Threads
- TreeSet
- LinkedHashSet
- HashMap
- TreeMap
- LinkedHashMap
- HashTable
- Iterator and ListIterator
- String
- Scanner
- Array Small Programs
- Introduction
- Variables
- DataTypes
- Operators
- Conditional Statements
- Loop
- Arrays
- Object Oriented Programming
- Classes and Objects
- Access Modifiers
- Methods
- Abstraction
- Constructor
- Packages
- StringBuffer
- Inheritance
- StringBuilder
- Encapsulation
- Java IO
- Polymorphism
- Interface
- Types Of Variables
- Static Keyword
- Final Keyword
- Super Keyword
- This Keyword
- Exception Handling